C Library
PHP Module
Perl Module
Ruby Library
Python Library
Apache Module
Nginx Module
Node.js Module
Cocoa / Objective C Library
Pascal Library
D Library
Go Package
Erlang Module
Haskell Package
Debian Package
Lua Package
Kotlin Module
Scala Library
OpenResty Package
Splunk Add-on
Apache Kafka Transform
OCaml Module
Deno Module
R Package
.NET Component
Java Component
HTTP Module
ActiveX / COM DLL
IP2Location IP Geolocation Scala Library
This IP Geolocation library is the Scala programming language library to support all IP2Location™ database products. It has been optimized for speed and memory utilization. Developers can use the module to query all IP2Location™ binary databases for applications written using the Scala programming language.
Sample Codes
object IP2LocationTest {
def main(args: Array[String]): Unit = {
try {
val ip = "8.8.8.8"
// querying with the BIN file
val loc = new IP2Location
val binfile = "/usr/data/IP-COUNTRY-REGION-CITY-LATITUDE-LONGITUDE-ZIPCODE-TIMEZONE-ISP-DOMAIN-NETSPEED-AREACODE-WEATHER-MOBILE-ELEVATION-USAGETYPE-ADDRESSTYPE-CATEGORY-DISTRICT-ASN.BIN"
val useMMF = true
loc.Open(binfile, useMMF)
val rec = loc.IPQuery(ip)
if ("OK" == rec.getStatus) System.out.println(rec)
else if ("EMPTY_IP_ADDRESS" == rec.getStatus) System.out.println("IP address cannot be blank.")
else if ("INVALID_IP_ADDRESS" == rec.getStatus) System.out.println("Invalid IP address.")
else if ("MISSING_FILE" == rec.getStatus) System.out.println("Invalid database path.")
else if ("IPV6_NOT_SUPPORTED" == rec.getStatus) System.out.println("This BIN does not contain IPv6 data.")
else System.out.println("Unknown error." + rec.getStatus)
loc.Close()
// querying with the web service
val ws = new IP2LocationWebService
val strAPIKey = "XXXXXXXXXX" // replace this with your IP2Location Web Service API key
val strPackage = "WS25"
val addOn = Array("continent", "country", "region", "city", "geotargeting", "country_groupings", "time_zone_info")
val strLang = "es"
val boolSSL = true
ws.Open(strAPIKey, strPackage, boolSSL)
var myresult = ws.IPQuery(ip, addOn, strLang)
if (myresult.get("response") == null) { // standard results
System.out.println("country_code: " + (if (myresult.get("country_code") != null) myresult.get("country_code").getAsString
else ""))
System.out.println("country_name: " + (if (myresult.get("country_name") != null) myresult.get("country_name").getAsString
else ""))
System.out.println("region_name: " + (if (myresult.get("region_name") != null) myresult.get("region_name").getAsString
else ""))
System.out.println("city_name: " + (if (myresult.get("city_name") != null) myresult.get("city_name").getAsString
else ""))
System.out.println("latitude: " + (if (myresult.get("latitude") != null) myresult.get("latitude").getAsString
else ""))
System.out.println("longitude: " + (if (myresult.get("longitude") != null) myresult.get("longitude").getAsString
else ""))
System.out.println("zip_code: " + (if (myresult.get("zip_code") != null) myresult.get("zip_code").getAsString
else ""))
System.out.println("time_zone: " + (if (myresult.get("time_zone") != null) myresult.get("time_zone").getAsString
else ""))
System.out.println("isp: " + (if (myresult.get("isp") != null) myresult.get("isp").getAsString
else ""))
System.out.println("domain: " + (if (myresult.get("domain") != null) myresult.get("domain").getAsString
else ""))
System.out.println("net_speed: " + (if (myresult.get("net_speed") != null) myresult.get("net_speed").getAsString
else ""))
System.out.println("idd_code: " + (if (myresult.get("idd_code") != null) myresult.get("idd_code").getAsString
else ""))
System.out.println("area_code: " + (if (myresult.get("area_code") != null) myresult.get("area_code").getAsString
else ""))
System.out.println("weather_station_code: " + (if (myresult.get("weather_station_code") != null) myresult.get("weather_station_code").getAsString
else ""))
System.out.println("weather_station_name: " + (if (myresult.get("weather_station_name") != null) myresult.get("weather_station_name").getAsString
else ""))
System.out.println("mcc: " + (if (myresult.get("mcc") != null) myresult.get("mcc").getAsString
else ""))
System.out.println("mnc: " + (if (myresult.get("mnc") != null) myresult.get("mnc").getAsString
else ""))
System.out.println("mobile_brand: " + (if (myresult.get("mobile_brand") != null) myresult.get("mobile_brand").getAsString
else ""))
System.out.println("elevation: " + (if (myresult.get("elevation") != null) myresult.get("elevation").getAsString
else ""))
System.out.println("usage_type: " + (if (myresult.get("usage_type") != null) myresult.get("usage_type").getAsString
else ""))
System.out.println("address_type: " + (if (myresult.get("address_type") != null) myresult.get("address_type").getAsString
else ""))
System.out.println("category: " + (if (myresult.get("category") != null) myresult.get("category").getAsString
else ""))
System.out.println("category_name: " + (if (myresult.get("category_name") != null) myresult.get("category_name").getAsString
else ""))
System.out.println("credits_consumed: " + (if (myresult.get("credits_consumed") != null) myresult.get("credits_consumed").getAsString
else ""))
// continent addon
if (myresult.get("continent") != null) {
val continentObj = myresult.getAsJsonObject("continent")
System.out.println("continent => name: " + continentObj.get("name").getAsString)
System.out.println("continent => code: " + continentObj.get("code").getAsString)
val myarr = continentObj.getAsJsonArray("hemisphere")
System.out.println("continent => hemisphere: " + myarr.toString)
System.out.println("continent => translations: " + continentObj.getAsJsonObject("translations").get(strLang).getAsString)
}
// country addon
if (myresult.get("country") != null) {
val countryObj = myresult.getAsJsonObject("country")
System.out.println("country => name: " + countryObj.get("name").getAsString)
System.out.println("country => alpha3_code: " + countryObj.get("alpha3_code").getAsString)
System.out.println("country => numeric_code: " + countryObj.get("numeric_code").getAsString)
System.out.println("country => demonym: " + countryObj.get("demonym").getAsString)
System.out.println("country => flag: " + countryObj.get("flag").getAsString)
System.out.println("country => capital: " + countryObj.get("capital").getAsString)
System.out.println("country => total_area: " + countryObj.get("total_area").getAsString)
System.out.println("country => population: " + countryObj.get("population").getAsString)
System.out.println("country => idd_code: " + countryObj.get("idd_code").getAsString)
System.out.println("country => tld: " + countryObj.get("tld").getAsString)
System.out.println("country => translations: " + countryObj.getAsJsonObject("translations").get(strLang).getAsString)
val currencyObj = countryObj.getAsJsonObject("currency")
System.out.println("country => currency => code: " + currencyObj.get("code").getAsString)
System.out.println("country => currency => name: " + currencyObj.get("name").getAsString)
System.out.println("country => currency => symbol: " + currencyObj.get("symbol").getAsString)
val languageObj = countryObj.getAsJsonObject("language")
System.out.println("country => language => code: " + languageObj.get("code").getAsString)
System.out.println("country => language => name: " + languageObj.get("name").getAsString)
}
// region addon
if (myresult.get("region") != null) {
val regionObj = myresult.getAsJsonObject("region")
System.out.println("region => name: " + regionObj.get("name").getAsString)
System.out.println("region => code: " + regionObj.get("code").getAsString)
System.out.println("region => translations: " + regionObj.getAsJsonObject("translations").get(strLang).getAsString)
}
// city addon
if (myresult.get("city") != null) {
val cityObj = myresult.getAsJsonObject("city")
System.out.println("city => name: " + cityObj.get("name").getAsString)
System.out.println("city => translations: " + cityObj.getAsJsonArray("translations").toString)
}
// geotargeting addon
if (myresult.get("geotargeting") != null) {
val geoObj = myresult.getAsJsonObject("geotargeting")
System.out.println("geotargeting => metro: " + geoObj.get("metro").getAsString)
}
// country_groupings addon
if (myresult.get("country_groupings") != null) {
val myarr = myresult.getAsJsonArray("country_groupings")
if (myarr.size > 0) for (x <- 0 until myarr.size) {
System.out.println("country_groupings => #" + x + " => acronym: " + myarr.get(x).getAsJsonObject.get("acronym").getAsString)
System.out.println("country_groupings => #" + x + " => name: " + myarr.get(x).getAsJsonObject.get("name").getAsString)
}
}
// time_zone_info addon
if (myresult.get("time_zone_info") != null) {
val tzObj = myresult.getAsJsonObject("time_zone_info")
System.out.println("time_zone_info => olson: " + tzObj.get("olson").getAsString)
System.out.println("time_zone_info => current_time: " + tzObj.get("current_time").getAsString)
System.out.println("time_zone_info => gmt_offset: " + tzObj.get("gmt_offset").getAsString)
System.out.println("time_zone_info => is_dst: " + tzObj.get("is_dst").getAsString)
System.out.println("time_zone_info => sunrise: " + tzObj.get("sunrise").getAsString)
System.out.println("time_zone_info => sunset: " + tzObj.get("sunset").getAsString)
}
}
else System.out.println("Error: " + myresult.get("response").getAsString)
myresult = ws.GetCredit
if (myresult.get("response") != null) System.out.println("Credit balance: " + myresult.get("response").getAsString)
} catch {
case e: Exception =>
System.out.println(e)
e.printStackTrace(System.out)
}
}
}
Sample IP2Location Databases (BIN)
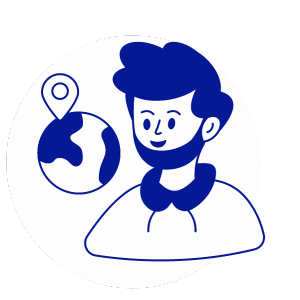
Discover Your User Locations
Retrieve geolocation data for FREE now!